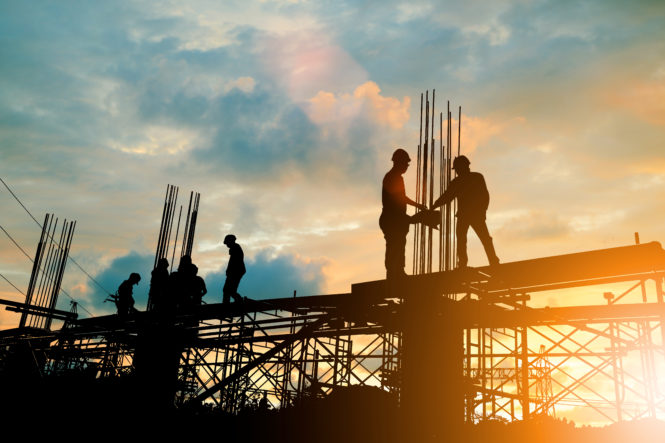
Venturing into Windows driver development can be an intimidating endeavor, especially if it’s your first time. You may find yourself asking, “Where do I start?” Comprehensive tutorials abound on creating drivers for the latest versions of Windows, but guidance on doing so for legacy systems can be more challenging to locate. This guide aims to provide clear, step-by-step instructions for customizing and building Windows Packet Filter drivers across all supported Windows platforms, ranging from Windows 95 to Windows 10.
The layout of the Windows Packet Filter source code.
Upon installing the Windows Packet Filter Source Code package, you’ll find a folder named kernel
in the main installation directory. The kernel
folder comprises:
/bin
: Houses driver binaries for all supported Windows versions./common
: Contains source code shared across all supported Windows versions. This folder should be your primary target for adding new code when constructing a kernel-mode solution based on Windows Packet Filter./IM
: Includes files pertinent to the NDIS 5.1 Intermediate driver build, which is employed on Windows XP and Windows Server 2003. This driver can also operate on several later Windows versions, but not on Windows 10./LWF
: Incorporates files specific to NDIS 6.x Lightweight Filter driver builds used from Windows Vista (NDIS 6.0) through to Windows 10 (NDIS 6.30)./ndisrdlwf Package
: Provides the NDIS 6.x Lightweight Filter driver companion Visual Studio 2012 project, primarily for building installation packages./SYS
: Includes files relating to Windows NT 4.0 and Windows 2000 NDIS-hooking drivers, which can also be used for 32-bit Windows XP/2003, but not on 64-bit builds due to PatchGuard./VxD
: Contains files specific to Windows 95, Windows 98, and Windows Millennium./ndisapi
: Carries the source code for the NDISAPI.DLL, which provides C/C++ interfaces to Windows Packet Filter drivers./INF
: Holds INF files needed for building installation packages for NDIS 5.1 Intermediate driver and NDIS 6.x Lightweight Filter driver.
Building drivers for legacy systems prerequisites
To build Windows Packet Filter drivers for legacy Windows versions, certain tools may be required. These might be hard to find in today’s age:
- For Windows 95, 98, and ME: Visual Studio 6.0 and VToolsD.
- For Windows NT 4.0 and Windows 2000: Microsoft Windows 2000 Driver Developer Kit.
- For Windows XP and Windows Server 2003: Driver Development Kits like Microsoft Windows Server 2003 Driver Developer Kit, Windows Vista WDK, or Windows 7 WDK.
Building driver for modern Windows systems prerequisites
For modern Windows systems, the construction of drivers requires different tools depending on the target operating system.
To build drivers for Windows Vista, you should use Microsoft Visual Studio 2012 and Windows 8 WDK. However, please bear in mind that there is currently no official method to sign drivers for Windows Vista, rendering the likelihood of utilizing these in a production environment quite low.
For systems running Windows 7 and beyond, you should utilize either Visual Studio 2017 or 2019 in conjunction with the Windows 10 WDK for driver construction. It’s important to note that while these tools are capable of building a wide range of drivers, they unfortunately do not support the construction of drivers for Windows Vista and Windows Server 2008.
Before the build process begins, it is imperative to select a new driver/device name in place of the standard NDISRD
, and generate a new GUID for NDIS 6.x Lightweight Filter driver. Code signing certificates from a Microsoft authorized CA authority are necessary for production builds due to driver code signing policy on 64-bit versions of Windows Vista and later. For Windows 10/11, an Extended Validation (EV) Code Signing certificate is required.
Once you’ve installed the certificates, Visual Studio can handle driver signing and driver packages generation processes, provided the correct certificates are configured in project settings. Windows 10, however, necessitates additional steps to sign the driver using the Windows Hardware Developer Center Dashboard portal.
Building Windows Packet Filter drivers
Part I. Common changes
To customize your driver/device name, navigate to the common.h file located in the /include
directory. Within this file, locate all instances of NDISRD
and ndisrd
. Replace these instances with your chosen new name for the driver/device:
// Common strings set
#define DRIVER_NAME_A "NDISRD"
#define DRIVER_NAME_U L"NDISRD"
#define DEVICE_NAME L"\\Device\\NDISRD"
#define SYMLINK_NAME L"\\DosDevices\\NDISRD"
#define WIN9X_REG_PARAM "System\\CurrentControlSet\\Services\\VxD\\ndisrd\\Parameters"
#define WINNT_REG_PARAM TEXT("SYSTEM\\CurrentControlSet\\Services\\ndisrd\\Parameters")
#define FILTER_FRIENDLY_NAME L"WinpkFilter NDIS LightWeight Filter"
#define FILTER_UNIQUE_NAME L"{CD75C963-E19F-4139-BC3B-14019EF72F19}" //unique name, quid name
#define FILTER_SERVICE_NAME L"NDISRD"
To further personalize your driver, begin by altering the FILTER_UNIQUE_NAME
in the common.h
file, located in the /include
directory. Substitute it with the new GUID (Globally Unique Identifier) that you’ve generated for your NDIS 6.x Lightweight Filter driver. For improved clarity, it’s recommended to replace FILTER_FRIENDLY_NAME
with a description that’s more indicative of your software.
The next step involves the INF files located in the kernel/INF directory. Start by renaming ndisrd.inf
, ndisrd_m.inf
, and ndisrd_lwf.inf
. Change ndisrd
in these filenames to the new name you’ve chosen for your driver/device. Following this, open each renamed INF file and replace all instances of ndisrd
with your new driver/device name.
Each INF file ends with a [Strings]
section where you can further customize descriptions to match your project better. For the renamed ndisrd_lwf.inf
file, an extra step is required. Find the [Install]
section and look for the string beginning with NetCfgInstanceId =
. Replace the GUID mentioned here with the one you’ve generated and added as the FILTER_UNIQUE_NAME
value in common.h
. This ensures that the GUID in the INF file corresponds to the GUID in common.h
, which is crucial for successful driver installation and operation.
Part II. Building NDIS 6.x Lightweight Filter drivers
The first adjustment to make involves the Visual Studio NDIS 6.x Lightweight Filter projects, specifically within the ndisrdlwf.vcxproj
(for Windows Vista/2008) and ndisrdlwf.2017.vcxproj
(for Windows 7/2008R2 and later) files. These are located in the /kernel/LWF
directory. To adapt these files for your new driver/device name, start by opening each of the mentioned files individually in a text editor. Substitute all instances of ndisrd
, ndisrd.cat
, and ndisrd_lwf.inf
with the driver/device name you have chosen. After implementing these changes, reload the revised projects in Visual Studio.
With the use of the Visual Studio resource editor, you can further customize the project version resource. You can add your company name, product name, and copyright information to this section for additional personalization.
The steps mentioned above apply to both Visual Studio 2012 with Windows 8 WDK, and Visual Studio 2017/2019 with Windows 10 WDK. However, subsequent steps will vary depending on your specific development environment and the target Windows version for your driver.
Using Visual Studio 2012 with Windows 8 WDK for Windows Vista/2008 targets
- Load both
ndisrdlwf.vcxproj
andndisrdlwf Package.vcxproj
projects into one workspace in Visual Studio 2012. - Configure your code signing certificates within the properties of both the
ndisrdlwf
andndisrdlwf Package
projects (navigate through: Configuration Properties → Driver Signing). For optimal results, it is advisable to specify a Test certificate for debug builds, and a production certificate for Release builds. - Proceed to rebuild all projects. During the rebuild process, Visual Studio 2012 will handle the building and signing of the driver binaries for
ndisrdlwf
. For thendisrdlwf Package
, it will generate and sign CAT (Catalog) files.
Using Visual Studio 2017/2019 with Windows 10 WDK for Windows 7 and later targets
- Unlike Visual Studio 2012 you don’t need
ndisrdlwf Project
here, so simply openndisrdlwf.2017.vcxproj
. - Configure your code signing certificates in
ndisrdlwf
project properties (Configuration Properties ⇾ Driver Signing). It is recommended to specify Test certificate for debug builds and production certificate for Release ones. - Rebuild the project’s configurations. Visual Studio will build and sign driver binaries and generate and sign CAT files as well.
Part III. Building NDIS 5.1 Intermediate driver
This type of driver is utilized for Windows XP and Windows Server 2003. It can be compiled using various toolsets, including the Microsoft Windows Server 2003 Driver Developer Kit, Windows Vista WDK, or the Windows 7 WDK.
- Go to
WinpkFilter/Kernel/IM
- Edit
ndisrd.rc
to include any company, product or copyright information into the version resource. - Open a file named
sources
and in the first stringTARGETNAME=ndisrd
replacendisrd
with the driver/device name you have chosen. - Start
Windows Server 2003 x64 Free Build Environment
and WindowsServer 2003 x86 Free Build Environment
. Each will open a new command line window. - In each command line window opened in previous step, change directory to
WinpkFilter/Kernel/IM
and rundbuild.bat
script.
Part IV. Building Windows NT 4.0 and Windows 2000 NDIS-hooking driver
This driver type is designed for Windows NT 4.0 and Windows 2000, and it can also be deployed on a 32-bit Windows XP system. The driver can be compiled using the Windows 2000 Driver Development Kit.
- Go to
WinpkFilter/Kernel/SYS
folder. - Edit
ndisrd.rc
to include any company, product or copyright information into the version resource. - Open a file named
sources
and in the first stringTARGETNAME=ndisrd
replacendisrd
with the driver/device name you have chosen. - Start Windows 200 Free Build Environment to open a new command line window.
- In command line window opened in previous step, change directory to
WinpkFilter/Kernel/SYS
and rundbuild.bat
script.
Part V. Building Windows 95, Windows 98, Windows Millennium Edition NDIS-hooking driver
This specific driver type is compatible with Windows 95, Windows 98, and Windows Millennium Edition. Building this driver will necessitate the use of certain legacy tools:
- Microsoft Visual Studio 6.0
- Compuware/Numega VToolsD (the latest release was included in Compuware Driver Studio 2.0)
To customize the driver name and resources please perform the steps below:
- Navigate to
WinpkFilter/Kernel/VxD
- Open
NDISRDr.mak
in text editor. Find the stringDEVICENAME = NDISRD
and changeNDISRD
to the driver/device name you have chosen. - Repeat the step above for
NDISRD.mak
. - Rename
NDISRD.VRC
toYOU_DRIVER_NAME.VRC
and edit this version resource to include any company, product or copyright information. - Open
w9xndisrd.dsp
in Microsoft Visual Studio 6.0 and rebuild the project.
Part VI. Conclusion
Conclusion:
This guide has offered a comprehensive walkthrough of the process of customizing and building Windows Packet Filter drivers across various Windows platforms and development environments. The steps outlined here provide the foundation needed to personalize and develop your own unique drivers, right from legacy Windows versions to the latest operating systems.
As you journey through the process, remember to adjust your approach based on your target Windows version and chosen development environment. Stay mindful of the unique considerations and challenges each platform presents, and adjust your approach accordingly.
Don’t hesitate to revisit this guide as often as needed. We hope it serves as a valuable resource in your driver development journey. Feel free to share your experiences, insights, or questions in the comments section below. Your contributions will certainly help others who are navigating a similar path.
We’re excited to see the innovative drivers you’ll develop. Happy coding!